|
|
BACK
Automatic
Feed Back Control On-Off for Temperature Chamber
- Microcontroller 8051
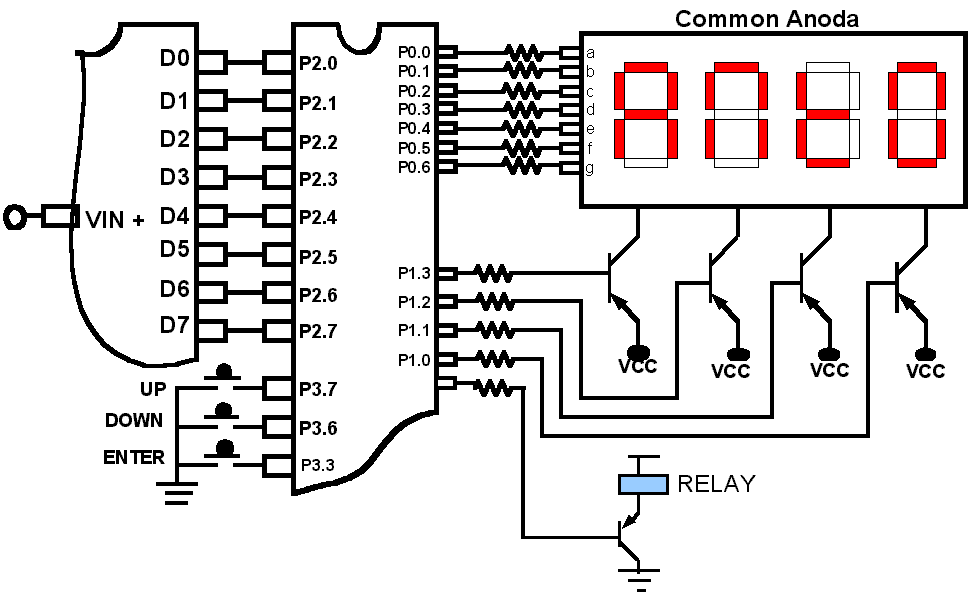
( Download File asm : feedback.zip , Temperature Regulation Circuit: temp.zip )
;*******************Copyright*****************************
; Triwiyanto -> info@mytutorialcafe.com ;================================================================
ADC_WR bit P1.5
ADC_INT bit P1.6
ControlBit bit 20h.0
DataADC equ 60h
DataSetting equ 61h
Settingone equ 65h
Settingten equ 66h
Settinghundred equ 67h
tempone equ 68h
tempten equ 69h
temphundred equ 6ah
;
counter20 equ 70h
counter60 equ 71h
minute equ 72h
hour equ 73h
minute1 equ 74h
minute10 equ 75h
hour1 equ 76h
hour10 equ 77h
second equ 78h
second1 equ 79h
second10 equ 7ah
org 0h
ljmp start
;==================================
; vektor interrupt location TF0 ->0Bh
;==================================
org 3h
reti
org 0bh
ajmp timerinterrupt
org 13h
reti
org 23h
reti
;
;============================================
;Subrutine to read data ADC and
;saving data ADC to dataADC
;============================================
ADC:
clr ADC_WR ; start of conversion
setb ADC_WR
not_EOC:
jb ADC_INT,not_EOC;wait end of conversion
mov a,p2
mov dataADC,a
ret
;
;=================================================================
;PROCEDURE CONTROL
;by comparing DATA ADC and DATA SETTING, and result will be output to bit P1.4
;===================================================================
Controltemp:
mov a,Datasetting
mov b,dataADC
clr c
subb a,b
jnz OnHeater
ret
OnHeater:
jc OffHeater
Clr p1.4 ; heater= on
Setb ControlBit
ret
OffHeater:
Setb p1.4 ; heater=off
Clr ControlBit
ret
;
;=================================================
;Subrutine to display time to Seven Segmen
;=================================================
Displaytime:
Setb P1.3
Setb P1.2
Setb P1.1
Setb P1.0
Mov DPTR,#Decoder7Segmen
mov A,second1
Movc A,@A+DPTR
Mov P0,A
Clr P1.0
Acall delay
;
Mov DPTR,#Decoder7Segmen
Mov A,second10
Movc A,@A+DPTR
Setb P1.0
Mov P0,A
Clr P1.1
Acall delay
;
Mov DPTR,#Decoder7Segmen
Mov A,minute1
Movc A,@A+DPTR
Setb P1.1
Mov P0,A
Clr P1.2
Acall delay
;
Mov DPTR,#Decoder7Segmen
Mov A,minute10
Movc A,@A+DPTR
Setb P1.2
Mov P0,A
Clr P1.3
Acall delay
ret
;
Displaytemp:
Setb P1.3
Setb P1.2
Setb P1.1
Setb P1.0
;
mov DPTR,#fraction
MOv A,DataADC
Movc A,@A+DPTR
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Mov P0,A
Clr P1.0
Acall delay
;
mov DPTR,#one
MOv A,DataADC
Movc A,@A+DPTR
Mov tempone,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.0
Mov P0,A
Clr P1.1
Acall delay
;
mov DPTR,#ten
MOv A,DataADC
Movc A,@A+DPTR
Mov tempten,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.1
Mov P0,A
Clr P1.2
Acall delay
;
mov DPTR,#hundred
MOv A,DataADC
Movc A,@A+DPTR
Mov temphundred,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.2
Mov P0,A
Clr P1.3
Acall delay
;
;==============================================================================
;Joining data in one variabel
;DataADC = temphundred*100 + tempten*10 + temp*1
;==============================================================================
mov a,tempten
mov b,#10d
mul ab
mov DataADC,a
mov a,temphundred
mov b,#100d
mul ab
add a,DataADC
add a,tempone
mov DataADC,a
ret
;
DisplaytempOff:
mov DPTR,#fraction
MOv A,DataADC
Movc A,@A+DPTR
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
;
mov DPTR,#one
MOv A,DataADC
Movc A,@A+DPTR
Mov tempone,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
;
mov DPTR,#ten
MOv A,DataADC
Movc A,@A+DPTR
Mov tempten,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
;
mov DPTR,#hundred
MOv A,DataADC
Movc A,@A+DPTR
Mov temphundred,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
;
;==============================================================================
;Joining data in one variabel
;DataADC = temphundred*100 + tempten*10 + tempone
;==============================================================================
mov a,tempten
mov b,#10d
mul ab
mov DataADC,a
mov a,temphundred
mov b,#100d
mul ab
add a,DataADC
add a,tempone
mov DataADC,a
ret
;
;=============================================
;Subrutine to display Setting to 7 Segmen
;=============================================
DisplaySetting:
Setb P1.3
Setb P1.2
Setb P1.1
Setb P1.0
Mov P0,#11000000b
Clr P1.0
Acall delay
;
Mov DPTR,#one
Mov A,DataSetting
Movc A,@A+DPTR
Mov Settingone,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.0
Mov P0,A
Clr P1.1
Acall delay
;
Mov DPTR,#ten
Mov A,DataSetting
Movc A,@A+DPTR
Mov Settingten,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.1
Mov P0,A
Clr P1.2
Acall delay
;
Mov DPTR,#hundred
Mov A,DataSetting
Movc A,@A+DPTR
Mov Settinghundred,A
Mov DPTR,#Decoder7Segmen
Movc A,@A+DPTR
Setb P1.2
Mov P0,A
Clr P1.3
Acall delay
;=========================================================
;Joining data setting in one variabel
;DataSetting = Settinghundred*100 + Settingten*10 + Settingone
;=========================================================
mov a,Settingten
mov b,#10d
mul ab
mov DataSetting,a
mov a,Settinghundred
mov b,#100d
mul ab
add a,DataSetting
add a,Settingone
mov DataSetting,a
ret
;
delay: mov R0,#0
delay1: mov R2,#0fh
djnz R2,$
djnz R0,delay1
ret
;
;======================================================
;Subrutine to display code nama
;=====================================================
;
codeDisplay:
Setb P1.3
Setb P1.2
Setb P1.1
Setb P1.0
Clr A
Movc A,@A+DPTR
Mov P0,A
Clr P1.0
Acall delay
inc DPTR
;
Clr A
Movc A,@A+DPTR
Setb P1.0
Mov P0,A
Clr P1.1
Acall delay
inc DPTR
;
Clr A
Movc A,@A+DPTR
Setb P1.1
Mov P0,A
Clr P1.2
Acall delay
inc DPTR
;
Clr A
Movc A,@A+DPTR
Setb P1.2
Mov P0,A
Clr P1.3
Acall delay
ret
;
MovingDisplay:
mov R7,#50
Lagi1: Mov DPTR,#codeKlp1
Acall codeDisplay
Djnz R7,Lagi1
jnb p3.3,wait11
;
mov R7,#50
Lagi2: Mov DPTR,#codeKlp2
Acall codeDisplay
Djnz R7,Lagi2
jnb p3.3,wait11
;
mov R7,#50
Lagi3: Mov DPTR,#codeKlp3
Acall codeDisplay
Djnz R7,Lagi3
jnb p3.3,wait11
;
mov R7,#50
Lagi4: Mov DPTR,#codeKlp4
Acall codeDisplay
Djnz R7,Lagi4
jnb p3.3,wait11
ret
;
wait11: ljmp wait1
;
;==============================================
;Subrutine to untuk inisialisasi kerja dari timer
;dengan menggunakan mode 1 timer 0 16 bit
;dan pengaktivan interrupt TF0
;==============================================
InitTimer:
clr ControlBit
mov counter20,#20
acall UpdateDisplay
mov TMOD,#00000001b
mov tl0,#0b0h
mov th0,#03ch
setb ET0
setb EA
setb TR0
ret
;
;====================================================
;This is the most importan subrutine, to serve interuption when
;TF0 Timer 0 Call
;this instruction is used to serve interruption every 0.05 second. or 50.000 usecond
; so data to be loaded is 65536- 50000 = 15536 d = 3CB0 h ;load B0h to TL1 and 3C to TH1
;In this subroutine counter20 will decrement until counter20=0, and this will happen every
;20 X 50000 uS = 20 X 0,05s = 1 s,if this happen then subroutine hourdigital will be call
;to increment data second, minute, atau hour
;==================================================== Timerinterrupt:
mov tl0,#0b0h
mov th0,#03ch
djnz counter20,Endinterrupt
mov counter20,#20
acall hourdigital
Endinterrupt:
reti
;
;=============================================================
;This Subrutine is to increment data for i hour digital
;second dan minute, if second = 60 then counter minute wil increment
;this subroutine will be call every second
;=============================================================
hourSetting:
dec second
mov a,#0
cjne a,second,UpdateDisplaySetting
mov second,#60
;
oneminuteSetting:
dec minute
mov A,#0
cjne A,minute,UpdateDisplaySetting
mov minute,#60
; setb P3.3; Mematikan beban
; ljmp endprocess
;
UpdateDisplaySetting:
mov a,second
mov b,#10
div ab
mov second1,b
mov second10,a
;
mov a,minute
mov b,#10
div ab
mov minute1,b
mov minute10,a
ret
;
;==========================================================
;This subroutine is used to scan menute and second ;byt using P3.6 and P3.7 ;========================================================= ScanSettinghour:
JNB P3.7,hourSetting
JNB P3.6,oneminuteSetting
JNB P3.3,wait2
ret
;
;===================================================================
;This Subrutine is used to scan setting data temperatur ;====================================================================
ScanSettingtemp:
mov R7,#1
Down: mov DataSetting,R7
acall DisplaySetting
jnb p3.3,wait3
jb P3.7,up
inc R7
acall delay
sjmp down
;
Up: Mov DataSetting,R7
acall DisplaySetting
jnb P3.3,wait3
jb p3.6,down
dec R7
acall delay
sjmp down
ret
;
hourdigital:
dec second
mov a,#0
cjne a,second,UpdateDisplay
;
cjne a,minute,next
Setb p1.4
ljmp endprocess
;
next:
mov second,#59
;
oneminute:
dec minute
mov A,#0
cjne A,minute,UpdateDisplay
mov minute,#0
;
UpdateDisplay:
mov a,second
mov b,#10
div ab
mov second1,b
mov second10,a
;
mov a,minute
mov b,#10
div ab
mov minute1,b
mov minute10,a
ret
;
Start:
acall MovingDisplay
jb p3.3,start
;
Wait1:
jnb p3.3, wait1
mov second,#60
mov minute,#60
;
Settingtime:
acall Scansettinghour
acall Displaytime
sjmp Settingtime
;
wait2:
jnb p3.3, wait2
;
Settingtemp:
acall ScanSettingtemp
sjmp Settingtemp
;
wait3:
jnb p3.3,wait3
;
StartOperating:
acall ADC
acall Displaytemp
acall Controltemp
jb ControlBit,StartOperating
;
acall InitTimer
;
Clr P1.4;Menghidupkan Beban
;
Forevertime:
Acall adc
Acall displaytempOff
Acall Controltemp
Acall Displaytime
jnb P3.3,startAgain
jnb P3.6,Forevertemp
sjmp Forevertime
Forevertemp:
acall adc
Acall Displaytemp
Acall Controltemp
jnb p3.3, startAgain
jnb P3.7,Forevertime
sjmp Forevertemp
;
StartAgain:
jnb p3.3,startAgain
clr TR0
clr ET0
clr EA
sjmp start
;
;=====================================================================
;What do you think, I am use for?
;=====================================================================
endprocess:
mov P0,#11000000b
Clr P1.3
Clr P1.2
Clr P1.1
Clr P1.0
jnb p3.3,startAgain
sjmp endprocess
;
;======================================================================
; L O O K U P T A B L E
; Decoder to Seven Segmen -> g f e d c b a
;======================================================================
Decoder7Segmen:
DB 11000000b,11111001b,10100100b,10110000b,10011001b
DB 10010010b,10000010b,11111000b,10000000b,10010000b
;
;======================================================================
; L O O K U P T A B L E
; temp = DataADC*100/255
;======================================================================
;
fraction:
db 0,4,8,2,6,0,4,7,1,5,9,3,7,1,5,9,3,7,1,5,8,2,6,0,4,8,2,6,0,4,8,2,5,9,3,7,1,5,9,3,7,1,5,9,3,6,0,4,8,2,6
db 0,4,8,2,6,0,4,7,1,5,9,3,7,1,5,9,3,7,1,5,8,2,6,0,4,8,2,6,0,4,8,2,5,9,3,7,1,5,9,3,7,1,5,9,3,6,0,4,8,2
db 6,0,4,8,2,6,0,4,7,1,5,9,3,7,1,5,9,3,7,1,5,8,2,6,0,4,8,2,6,0,4,8,2,5,9,3,7,1,5,9,3,7,1,5,9,3,6,0,4,8
db 2,6,0,4,8,2,6,0,4,7,1,5,9,3,7,1,5,9,3,7,1,5,8,2,6,0,4,8,2,6,0,4,8,2,5,9,3,7,1,5,9,3,7,1,5,9,3,6,0,4
db 8,2,6,0,4,8,2,6,0,4,7,1,5,9,3,7,1,5,9,3,7,1,5,8,2,6,0,4,8,2,6,0,4,8,2,5,9,3,7,1,5,9,3,7,1,5,9,3,6,0,4,8,2,6,0
;
one:
db 0,0,0,1,1,2,2,2,3,3,3,4,4,5,5,5,6,6,7,7,7,8,8,9,9,9,0,0,1,1,1,2,2,2,3,3,4,4,4,5,5,6,6,6,7,7,8,8,8,9,9
db 0,0,0,1,1,2,2,2,3,3,3,4,4,5,5,5,6,6,7,7,7,8,8,9,9,9,0,0,1,1,1,2,2,2,3,3,4,4,4,5,5,6,6,6,7,7,8,8,8,9
db 9,0,0,0,1,1,2,2,2,3,3,3,4,4,5,5,5,6,6,7,7,7,8,8,9,9,9,0,0,1,1,1,2,2,2,3,3,4,4,4,5,5,6,6,6,7,7,8,8,8
db 9,9,0,0,0,1,1,2,2,2,3,3,3,4,4,5,5,5,6,6,7,7,7,8,8,9,9,9,0,0,1,1,1,2,2,2,3,3,4,4,4,5,5,6,6,6,7,7,8,8
db 8,9,9,0,0,0,1,1,2,2,2,3,3,3,4,4,5,5,5,6,6,7,7,7,8,8,9,9,9,0,0,1,1,1,2,2,2,3,3,4,4,4,5,5,6,6,6,7,7,8,8,8,9,9,0
;
ten:
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1
db 2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3
db 3,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5,5
db 5,5,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,6,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7,7
db 7,7,7,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,8,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,0
;
hundred:
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0
db 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1
;
codeKlp1:
DB 10100011b,10000111b,10101011b,10001000b
codeKlp2:
DB 10001000b,10100011b,10000111b,10101011b
codeKlp3:
DB 10101011b,10001000b,10100011b,10000111b
codeKlp4:
DB 10000111b,10101011b,10001000b,10100011b
end
|
|
Lesson 1:
T
o o l
1.1. Programmer
1.2. Edsim
51
1.3. MIDE-51
1.4. ATMEL
ISP
Lesson 2:
Input Output
2.1.LED
2.2.Swicht
2.3.7
Segmen
2.4.LCD
Character
2.5.ADC
2.6.DAC
2.7.Motor
Stepper
2.8.Keypad
Lesson 3:
Timer Counter
3.1.Basic
3.2.Mode
0
3.3.Mode
1
3.4.Mode
2
3.5.Mode
3
Lesson 4:
Serial Comm.
4.1.Basic
4.2.LED
4.3.Rotate
LED
4.2 ADC
4.3.LCD
Lesson 5:
Interuption
5.1.Basic
5.2.Timer
5.2.External
|